さまざまなデータ構造をC++で書いてみる(2)
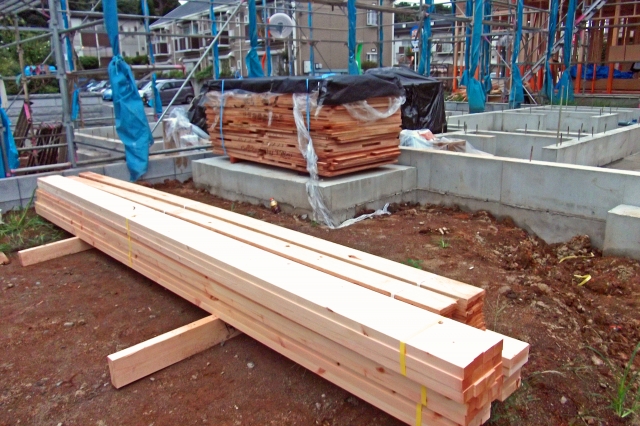
こんにちは。
前回の続きでさまざまなデータ構造をC++で書いてみます。
スタックをC++で書いてみる
以前の記事です。
それでは,2つ目の方の記事にあるプログラムをC++に直します。
わざわざ自分で書かなくてもすでにライブラリがあるというツッコミはしなくていいですよ。
ヘッダファイルのファイル名は mystack.h としておきます。
#include"MyLinkedList.h"
#ifndef _MYSTACK_H_
#define _MYSTACK_H_
#endif
#include <string>
using namespace std;
class MyStack
{
public:
MyStack();
bool empty();
string peek();
string pop();
void push(string);
string toString();
private:
MyLinkedList* myList;
};
次にクラス定義のファイルになります。
ファイル名は mystack.cpp にしておいてください。
#include "MyStack.h"
#include<iostream>
MyStack::MyStack()
{
this->myList = new MyLinkedList();
}
bool MyStack::empty()
{
return this->myList->size() == 0;
}
string MyStack::peek()
{
return this->myList->get(0);
}
string MyStack::pop()
{
string result = this->peek();
this->myList->remove(0);
return result;
}
void MyStack::push(string element)
{
this->myList->add(0, element);
}
string MyStack::toString()
{
return this->myList->toString();
}
これらの動作確認をするプログラムです。
#include<iostream>
#include"MyStack.h"
using namespace std;
int main() {
MyStack* st = new MyStack();
cout << "is empty? " << st->empty() << endl;
st->push("8");
cout << st->toString() << endl;
cout << "is empty? " << st->empty() << endl;
st->push("5");
cout << st->toString() << endl;
st->push("2");
cout << st->toString() << endl;
string s = st->pop();
cout << s << endl;
cout << st->toString() << endl;
cout << st->peek() << endl;
}
実行結果です。
is empty? 1
[8]
is empty? 0
[5] → [8]
[2] → [5] → [8]
2
[5] → [8]
5
キューをC++で書いてみる
続いてキューです。
こちらも以前の記事へのリンクをはっておきます。
こちらも同じようにc++に直します。
ヘッダファイルのファイル名は myqueue.h にしておきます。
#include"MyLinkedList.h"
#ifndef _MYQUEUE_H_
#define _MYQUEUE_H_
#endif
#include<iostream>
using namespace std;
class MyQueue
{
public:
MyQueue();
void offer(string);
string peek();
string poll();
bool isEmpty();
string toString();
private:
MyLinkedList* myList;
};
次にクラス定義のファイルになります。
ファイル名は myqueue.cpp にしておいてください。
#include "MyQueue.h"
#include<iostream>
MyQueue::MyQueue()
{
this->myList = new MyLinkedList();
}
void MyQueue::offer(string element)
{
this->myList->add(0, element);
}
string MyQueue::peek()
{
return this->myList->get(this->myList->size() - 1);
}
string MyQueue::poll()
{
string result = this->peek();
this->myList->remove(this->myList->size() - 1);
return result;
}
bool MyQueue::isEmpty()
{
return this->myList->size() == 0;
}
string MyQueue::toString()
{
return this->myList->toString();
}
キューが正しく動作しているかを確認するプログラムです。
#include<iostream>
#include"MyQueue.h"
using namespace std;
int main() {
MyQueue* qu = new MyQueue();
cout << "is empty? " << qu->isEmpty() << endl;
qu->offer("睦月");
cout << qu->toString() << endl;
cout << "is empty? " << qu->isEmpty() << endl;
qu->offer("如月");
cout << qu->toString() << endl;
qu->offer("弥生");
cout << qu->toString() << endl;
qu->offer("卯月");
cout << qu->toString() << endl;
string s = qu->peek();
cout << s << endl;
cout << qu->toString() << endl;
s = qu->poll();
cout << s << endl;
cout << qu->toString() << endl;
cout << qu->peek() << endl;
}
実行結果です。
is empty? 1
[睦月]
is empty? 0
[如月] → [睦月]
[弥生] → [如月] → [睦月]
[卯月] → [弥生] → [如月] → [睦月]
睦月
[卯月] → [弥生] → [如月] → [睦月]
睦月
[卯月] → [弥生] → [如月]
如月
今回はこれでおしまいにします。それでは,また。
ディスカッション
コメント一覧
まだ、コメントがありません